Recently, I had to implement personalization of Sitecore components based on third-party integrations and I decided to share one way of doing this. I say one way, because there are many ways to accomplish this, but here is one that worked well for me.
My goal was to help content authors edit a rule using the rule editor that would allow them to select an item from a list of items that needed to be displayed on a page. Also, the fields of the items from this list mapped to properties of an API response (i.e. whether the application is supposed to be shown or not). How the items are displayed are personalized based on the API’s response.
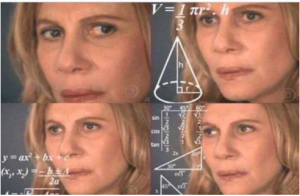
Complicated? Let me break this down then.
So, on one side we have a content author that wants to personalize the content based on a list selection. They have a list of application items and that’s how they determine which items to show on a page. Their goal is to select a few items from this list and have the content showing only for the applications selected and based on the application status being approved.
On the other side, we have an API which will receive a request based on a user and the application ID and will return the application status for that user. In this case, the status “approved” or “denied” are determined by the API.
To implement such personalization you can implement a custom rule which integrates with your API and returns the results based on the API response. With that response you can define your personalization(for example hiding a rendering or changing the datasource item based on the response). Ok, but how can I apply such personalization?
Here’s how:
Step 1
Create the list of application items. The items from the application list are instances of the same template (Application). We need to verify that on the Application template there is an “Application Id” field which we will use later to compare against the API response. Here is a sample item with the Application Id field set:

Step 2
Next we need to define a new rule element. To do so, navigate to the path “/sitecore/system/settings/rules/definitions/elements” and create a new element.
After creating the element (in this example “My Custom Element”) you also need to create a new condition. When you create a new condition, you get to specify how content authors will set the rules and you need to specify a type which implements that condition. If you are looking for more information on how to create custom conditions, this is an interesting reading.
In our case, the condition was defined like this:

The Text field is how one can define the text that the content authors see when adding the personalization through the rule editor. Looking at the screenshot above notice that there are four parameters on the conditions I created:
a – applicationid: this is the name of the property which we need to declare in our custom condition class.
b- Tree: I have used the Tree macro to specify that content authors can pick an item from a content tree.
c- root=/sitecore/content/MySite/Applications: As you probably figured this is the path as to where content authors will select the application from
d- application: This is the word that will appear on the rule editor for content authors to select the application
The Type field is where we define our custom condition implementation. Please refer to step 4 below.
Step 3
One other thing you will need to do is enable your condition within the Tags element. You can assign custom Tags to make the condition available as you can see here. But, for the sake of this post I’m keeping it simple and I’ll just use the Security Tag on the Taxonomy field which comes out-of-the-box with Sitecore.
Step 4
Previously, we have defined the condition and that condition had a field Type. Here is how you would implement it:
1 | namespace MyNamespace.Data.Rules |
3 | public class ApprovedApplication<T> : WhenCondition<T> where T: RuleContext |
7 | public string ApplicationId { get; set; } |
9 | private ContentService contentService; |
11 | public ContentService ContentService |
15 | if (contentService == null ) |
16 | contentService = new ContentService(); |
19 | set { contentService = value; } |
22 | protected override bool Execute(T ruleContext) |
24 | if (String.IsNullOrWhiteSpace(ApplicationId)) |
27 | var id = new ID(ApplicationId); |
31 | var applicationItem = Context.Database.GetItem(id); |
32 | if (applicationItem == null || |
33 | String.IsNullOrWhiteSpace(applicationItem[Constants.TemplateFieldNames.Application.APPLICATION_ID])) |
37 | var appId = applicationItem[Constants.TemplateFieldNames.Application.APPLICATION_ID]; |
39 | var userId = new UserService().GetCurrentUserName(); |
40 | if (string.IsNullOrWhiteSpace(userId)) |
44 | var app = new MyApiService().GetApplicationAccess(userId, appId); |
45 | if (app == null || app.AccessStatus != Constants.ToolAccessStatuses.APPROVED) |
Here is what this code does in a nutshell. When a rule is used to create personalization, the rule validates whether the application is selected, whether it can retrieve the Application ID, calls the API integration and checks that the status is approved.
Step 5
In the previous step, we showed that during the execution of the rule we had a call to the service:
1 | var app = new MyApiService().GetApplicationAccess(userId, appId); |
This service call has the implementation of the third-party API and the request / response look something like this:
Request:
We pass to the applications API the user “myUser” which should have access to a few applications including application 123456:
http://hostname/myapi/applications/myUser
Response:
The API returns on a JSON format the applications that the user has access to:
{
“user”: “myUser”,
“access”: {
“applicationId”: “123456”,
“requestedDate”: “2018-05-02T16:13:29.000Z”,
“status”: “Approved”
}
Now, all we have to do is de-serialize the response into an appropriate object.
Step 6
Now we need to test it. So I opened the item I wanted to apply personalization and created a personalization for a rendering in which I wanted to apply such a rule. In the rule editor, I was selected my custom rule and had to select the application I wanted to show on the page, and see that after the rule was in place, I could see it being driven by the response from the API. In other words the applications would only show on the page if the API response returned the status approved for such application.

And as with all things Sitecore, there is always more than one way to skin a cat. I hope this personalization tip using third party integrations will work for you.
Happy Coding! Until next time – Diego
Hi, I have a few questions regarding some basics in Sitecore.
Ex. I wan to know how to add a js tracking snippet in the head and also add extra js snippets in the footer.
Also few questions regarding adding the value of the JS API call as the segment ids for personalization.
Thanks in advance.