I will make a short break in my series about YouTube Video Picker. This one is worth it.
MVC Pipelines
You have probably seen Alex Shyba’s post on how to cascade renderings in Sitecore 6.5. It’s a neat solution with a little twist to the insertRenderings
pipeline (called as part of renderLayout
). It’s great but it doesn’t work on MVC layouts and renderings. Just go ahead and try it if you have a minute to spare. The Page Editor still uses executePageEditorAction
pipeline and it still manages field values the way it used to but the page itself renders differently. In short (and I will sure write more about it later), renderLayout
is not called for MVC layouts.
Please welcome Sitecore.Mvc.config
and Sitecore.Mvc.ExperienceEditor.config
(from App_ConfigInclude
). The pipeline that we will need to tap into to cascade MVC renderings is defined as:
1 | <mvc.getXmlBasedLayoutDefinition> |
2 | <processor type="[...] GetXmlBasedLayoutDefinition.GetFromLayoutField [...]"/> |
3 | </mvc.getXmlBasedLayoutDefinition> |
It is further extended for the Page Editor with:
1 | <mvc.getXmlBasedLayoutDefinition> |
3 | type="[...] GetXmlBasedLayoutDefinition.GetPageDesigningLayout [...]" |
4 | patch:before="processor[1]"/> |
6 | type="[...] GetXmlBasedLayoutDefinition.SetLayoutContext [...]" |
7 | patch:after="processor[@type='[...] GetXmlBasedLayoutDefinition.GetFromLayoutField [...]']"/> |
8 | </mvc.getXmlBasedLayoutDefinition> |
The default processor gets renderings for a page from the Layout Details field. The page editor will intervene and get it from the being edited state if one exists. We need to enrich the renderings with the ones cascaded down from the item’s parent so our trick will be to:
1 | <mvc.getXmlBasedLayoutDefinition> |
3 | patch:before="*[last()]" |
4 | type="[...] AddCascadedRenderings [...]"/> |
5 | </mvc.getXmlBasedLayoutDefinition> |
Almost there it feels like.
Layout Definition and Layout Deltas
The new MVC pipelines are lean, concise, terse. They don’t carry args.Renderings
anymore. They stick to bare metal – XElement
of the item’s Layout Definition
. In a simple form it’s:
1 | XDocument.Parse(item[FieldIDs.LayoutField]).Root; |
It’s not that simple though. Since layout deltas were introduced in 6.4 an item’s __Renderings
will have either a layout delta (in case presentation details were defined on the item’s template’s standard values) or the actual layout definition. Sitecore handles the split and merge for you. A much closer version is this then:
1 | XDocument.Parse(LayoutField.GetFieldValue(item.Fields[FieldIDs.LayoutField])).Root |
XmlDeltas
is worth looking into. That’s what LayoutField.GetFieldValue()
calls behind the scenes. And you may also want to read this nice blog post about how you can leverage layout deltas to build complex layout hierarchies.
Cascading Renderings
Now we’re ready to cascade some renderings. Firs off, a template that inherits from Standard Rendering Parameters. With its help we can now tell our renderings to cascade:
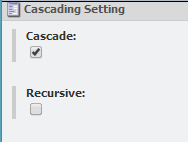
And a new AddCascadedRenderings
processor into mvc.getXmlBasedLayoutDefinition
:
[su_note note_color=”#fafafa”]Disclaimer: The code is experimental. It works but has certain limitations that I will get into and it has not been tested on real use cases.
Use at your own risk, as always.[/su_note]
The starting points:
1 | public class AddCascadedRenderings : GetXmlBasedLayoutDefinitionProcessor |
The pipeline handler:
1 | public override void Process(GetXmlBasedLayoutDefinitionArgs args) |
3 | if (args.Result == null ) return ; |
5 | Item item = PageContext.Current.Item; |
6 | Device device = PageContext.Current.Device; |
7 | if (item == null || item.Parent == null || device == null ) return ; |
9 | args.Result = MergeWithCascadedRenderings(args.Result, item.Parent, device); |
11 | Log.Debug( string .Format( "The result of merge is:n{0}" , args.Result)); |
My first stab at cascading renderings (i.e. add rendering nodes from the item’s direct parent’s presentation details that have the Cascade checkbox checked) was to manipulate the XMLs directly. It worked and I learned quite a bit about the differences between the deltas and full layouts but I ended up with a cleaner and a better solution:
1 | protected virtual XElement MergeWithCascadedRenderings(XElement self, Item parent, Device device) |
3 | Field layoutField = parent.Fields[FieldIDs.LayoutField]; |
5 | LayoutDefinition parentParsed = LayoutDefinition.Parse(LayoutField.GetFieldValue(layoutField)); |
6 | LayoutDefinition selfParsed = LayoutDefinition.Parse(self.ToString()); |
8 | Log.Debug( string .Format( "Merging n{0} nwithn {1}" , selfParsed.ToXml(), parentParsed.ToXml())); |
10 | string deviceId = string .Format( "{{{0}}}" , device.Id.ToString().ToUpper()); |
12 | DeviceDefinition selfDevice = selfParsed.GetDevice(deviceId); |
13 | DeviceDefinition parentDevice = parentParsed.GetDevice(deviceId); |
15 | if (parentDevice.Renderings == null ) return self; |
17 | parentDevice.Renderings.Cast<RenderingDefinition>() |
18 | .Where(r => StringUtil.ExtractParameter( "Cascade" , r.Parameters ?? "" ) == "1" ) |
19 | .ForEach(selfDevice.AddRendering); |
21 | return XDocument.Parse(selfParsed.ToXml()).Root; |
With the two definitions we get a hold of the same device within each one and then search for all renderings in the parent’s that need to cascade. All that are found are added to the current item.
Limitations
- This solution only cascades down to the immediate children. You will see in your DEBUG logs that the resulting layout carries over the Cascade parameter but that rendering is truly virtual. The rendering engine runs it but Page Editor doesn’t know it’s there. Well, it does, and that’s the next limitation, but it won’t save it on the item. You can confirm in your browser’s JS console that
$sc('#scLayout')
‘s JSON doesn’t know about the rendering that cascaded out of the blue. Like I said in the beginning, Sitecore’s mechanisms to fetch the item’s data for edit is pretty much the same. It’s the rendering that changed with MVC - While Page Editor doesn’t record the cascaded rendering on the item it has it rendered. The HTML markup is there. You can add new renderings before the cascaded one. You can’t add them after. Doing so will result in an error. I am yet to dig into it but I suspect it’s the position that confuses Sitecore. That POST to
Pallete.aspx
will tell Sitecore to insert the new rendering in the +1 position to what it knows exists. - Page Editor will allow interacting with the cascaded layout as if it was the item’s. But it’s not.
A more robust solution would disable Page Editor interactivity for the cascaded renderings and it would also allow to cascade further down the content tree unless the inheritance stopped. Pretty much like Adobe CQ’s iparsys. But’s that’s another story. Next time I will continue the YoutTube Video Picker series.
[su_divider][/su_divider]
One last thing. Enable pipelines profiling with Sitecore.PipelineProfiling.config
and look at your /sitecore/admin/pipelines.aspx
. And this blog post from John West makes a lot of sense wen you need to debug into a pipeline.
Hi Pavel,
Did you ever find a solution to the limitation of not being able to add components after the cascaded one?
Best regards,
Ben.
Hi Ben,
This blog post was just an experiment. We now have a full support for cascades in SCORE. We no longer cascade individual renderings either, we now cascade placeholders. You can see it in the recent virtual user group Brian and I did. We haven’t yet recorded a video about cascades on our SCORE YouTube Channel but I am sure it’s coming up.
We don’t allow to insert renderings after the cascade through, only before. The cascaded group of components will be basically pushed down in the receiving placeholder. It can be worked around – I think I know what it would take in our current implementation – but we decided that it wasn’t worth the effort. Cascading placeholders is more powerful than cascading individual renderings and we also have snippets (another feature of SCORE that we ought to record a short video about but you will see it demoed in that user group talk I mentioned) so I guess allowing to insert components after a cascade was not something we wanted hard enough.
Pavel
Hi Pavel,
Thanks for your reply. Inserting renderings after the cascade is exactly what I do need, so guess I will have to keep on digging in to what’s happening 🙂
Best regards,
Ben.
> We’re having the same issue now and came across this, did you manage to get a Solution to this at all Ben?