It is easier to test MultipartFile upload service in POSTMAN just by selecting multiple files and sending the request. But to test using Java, we need to write multiple lines of code to invoke the request. In this blog, we will discuss about the Java function to invoke MultipartFile upload request and get the response.
So, below are the steps carried out to upload the file in the request.
- Convert the file to MultipartFile
- GetByteArray of the multipart file
- Invoke request by adding the byteArray to body of the request
For instance, let us take the below example of File upload service. This service allows us to select n number of files and gives the count of the files as response
Method: POST
Url : http://localhost:8080/upload
Body :
Key : file
Value : (Select files from system)
Response : 200 OK
Response body: Count of the files attached
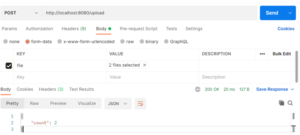
Attach MultipartFile in POSTMAN
Step 1: Convert the file to MultipartFile
Firstly, we need to convert the file to multipartFile. I have used a sample json file “requestBody1.json”. It is placed under “src/test/resources” in the Java project. The below function converts the file to multipart file using MockMultipartFile with filename, contentType, content which has file as bytes.
Following are the Parameters used by the function
filename = “requestBody1.json”.
filePath= “src/test/resources”.
contentType= “application/json”.
Following are the Imports used by the function
import org.springframework.web.multipart.MultipartFile;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import org.springframework.mock.web.MockMultipartFile;
Below is the function which converts the file to MultipartFile
public MultipartFile getMultipartFile(String fileName) {
Path path=Paths.get(filePath);
String name= fileName;
String originalFileName = fileName;
String contType = contentType;
byte[] content = null;
try {
content = Files.readAllBytes(path);
}catch (final IOException e) {}
MultipartFile result = new MockMultipartFile(name, originalFileName, contType, content);
return result;
}
Step 2: GetByteArray of the MultipartFile
Secondly, after converting the file to multipartFile, we need to get the byteArray of the multipartfile to add it to the body, so that we can invoke the request with the files.
Following are the Parameters used by the function
filename = “requestBody1.json”
contentType= “application/json”
parameterName=”file” (please refer postman screenshot to find the parameter name)
Following are the Imports used by the function
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.http.HttpEntity;
Below is the function to get the multipartFile in ByteArray
public HttpEntity<byte[]> getFileInByteArray(String fileName) throws IOException{
MultipartFile file=getMultipartFile(fileName);
//Get byteArray of the file
LinkedMultiValueMap<String,String> headerMap = new LinkedMultiValueMap<>();
headerMap.add(“Content-disposition”, “form-data; name=” + parameterName + “; filename=” +file.getOriginalFilename());
headerMap.add(“Content-type”, contentType);
HttpEntity<byte[]> doc = new HttpEntity<byte[]>(file.getBytes(),headerMap);
return doc;
}
Step 3: Invoke MultipartFile request
Thirdly, add the byteArray of the file to the body of the request. First, declare the body as MultiValueMap. Then, add the byteArray which we got from previous two functions to the body. After that, set the content type of the header to Multipart form data. Finally, add body and headers to the HttpEntity.
Below is the function to invoke the multipartFile upload Request
MultiValueMap<String,Object> body=new LinkedMultiValueMap();
//Add file in ByteArray to body
body.add(parameterName, getFileInByteArray(file));
//Set the headers
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.MULTIPART_FORM_DATA);
//Add body and headers to HttpEntity
HttpEntity<MultiValueMap<String, Object>> entity= new HttpEntity<>(body,headers);
//Post the request
ResponseEntity<String> response=new RestTemplate().postForEntity(“http://localhost:8080/upload”, entity, String.class);
Step 4: Response Verification
The response we get by invoking the request is stored as ResponseEntity. Finally, we can verify the response code and the count of the files attached. Converting the response to JSONObject (org.json) will make the response validation easier. For the example we have used, we are verifying the response code to be 200 and count to be 2.
assertEquals(true,200==response.getStatusCodeValue());
JSONObject obj= new JSONObject(response.getBody());
int cnt=obj.getInt(“count”);
assertEquals(true,cnt==2);
Conclusion
In this blog, you have successfully attached the files in the request and validated the response.
The following github url gives the testing code using cucumber framework
https://github.com/lav16kosh/test-multipartFile-upload-api.git